Name:
Actor Visualizer
Description:
Add support for Actor Visualizers, to compliment the existing Component Visualizers present in Unreal. Not all functionality is present in a component, making visualisation a pain. With this plugin, simply author a new FActorVisualiser, register it to the FActorVisualizeModule, and have it be rendered whenever an actor of interest is selected. Supports in world primitive drawing, as well as drawing directly to the HUD canvas.
Target Versions:
UE4.27, UE5.0, UE5.1
Target Platforms:
Component visualizers are a fantastic compliment to active development. However, they require functionality to live within a child of UActorComponent. In stripped back codebases that have functionality directly in the base Actor class, it can be hard, if not impossible, to visualize params in editor easily.
This plugin adds the FActorVisualiser base class, that mirrors the existing FComponentVisualiser class (with some notable exclusions). Simply extend from this class, override it’s DrawVisualization and/or DrawVisualizationHUD functions to author custom visuals when selecting an actor. Once a visual has been authored, it can be registered to the ActorVisualizer module to be rendered in-viewport.
Features:
- Easy to use Native namespace for loading paths and classes in C++
- Blueprint function library allowing for global loads outside of native
Code Modules:
ActorVisualiser - Editor
Number of Blueprints: 0
Number of C++ Classes: 4
Network Replicated: No, system is editor only
Instructions
The Actor Visualizer can simply be used directly from the Unreal Marketplace.
To author a new visualizer, simply extend off of the FActorVisualizer class, provided in this plugin. Here is an example of a generic visualizer that will render both in world and onto the screen -
An example of a visualiser with in world and HUD rendering
#pragma once
#include <ActorVisualizer/Public/Visualizer/ActorVisualizer.h>
#include <Runtime/Engine/Public/CanvasItem.h>
class FDefaultActorVisualizer : public FActorVisualizer
{
public:
void DrawVisualization(const AActor& InActor, const FSceneView& InView, FPrimitiveDrawInterface& InPDI) override
{
//Draw a cube at world origin
DrawWireBox(&InPDI, FBox(FVector(-100, -100, -100), FVector(100, 100, 100)), FColorList::Grey, 1, 10.f);
}
void DrawVisualizationHUD(const AActor& InActor, const FViewport& InViewport, const FSceneView& InView, FCanvas& InCanvas) override
{
//Draw the actor's name at actor location in world space
FVector2D PixelLocation;
if (InView.WorldToPixel(InActor.GetActorLocation(), PixelLocation))
{
FCanvasTextItem TextItem(
PixelLocation,
FText::FromString(FString::Printf(TEXT("%s selected"), *InActor.GetName())),
GEngine->GetLargeFont(),
FLinearColor::White);
TextItem.Scale = FVector2D::UnitVector;
TextItem.EnableShadow(FLinearColor::Black);
TextItem.Draw(&InCanvas);
}
}
};
Once we've authored a visualiser outlining information that we want, we need to let the ActorVisualizer module know about it.
Firstly, this has to be called from AN EDITOR MODULE. Pushing this in a shipping build will result in compilation/linking errors; an ideal place for these to be registered is in the StartupModule of an editor only module, like so -
Inside the .cpp of an editor module
virtual void StartupModule() override
{
FActorVisualizerModule::RegisterActorVisualiser(AActor::StaticClass(), MakeShared<FDefaultActorVisualizer>());
}
The first parameter is the actor class we want to visualise, while the second param is a shared ptr to the visualiser class; in this instance, we want to use the DefaultActorVisualizer class we created above.
Every time we select something that matches the class we pass in (any Actor children), both the DrawVisualization and DrawVisualizationHUD are run for the appropriate visualizers.
Here's an example of what the above renders -
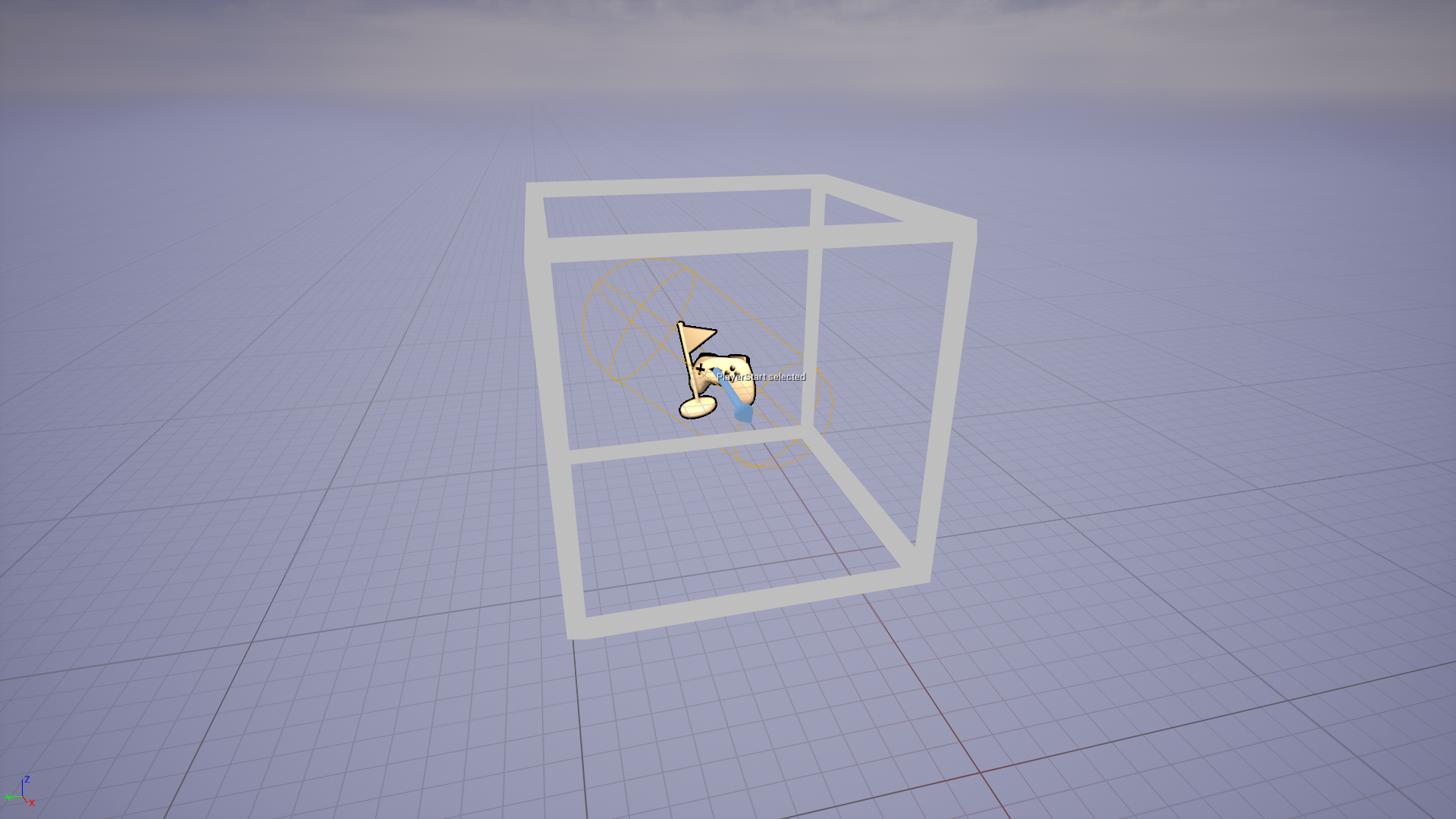
Support
This system leans heavily on Epic's own ComponentVisualizer API, so please make sure to check out their documentation here -
There's also an excellent post by Sondre Utheim on best practices regarding visualisers, especially primitive rendering -